Animate()는 다양한 효과 연출을 위해 다양한 기능을 제공한다.
1. 기본 사용법
animate의 문법은 아래와 같다.
$(selector).animate({params},speed,callback);
param : 애니메이션으로 정의할 CSS 속성.
speed : 애니메이션 효과 연출 시간
callback : 연출 종료 후 호출할 함수
아래는 animate()로 박스를 좌 <-> 우 로 움직인다.
<div id="box" style="background-color:pink; width:100px; height:100px;position:absolute;">눌러보세요</div>
var b = false;
$(document).ready(function(){
$("#box").click(function(){
if(!b) {$('#box').animate({left: '250px'}); b=!b;}
else { $('#box').animate({left: '0px'}); b=!b;}
});
});
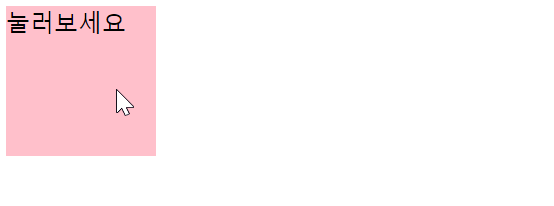
2. Animate 응용
Animate는 여러 속성을 동시에 적용이 가능하다.
아래의 코드는 박스가 커지며 색이 옅어지는 효과를 준다.
$(document).ready(function(){
$("#box2").click(function(){
$("#box2").animate({
left: '250px',
opacity: '0.5',
height: '150px',
width: '150px'
});
});
});
<div id="box2" style="background-color:skyblue; width:200px; height:200px;position:absolute;">눌러보세요</div>
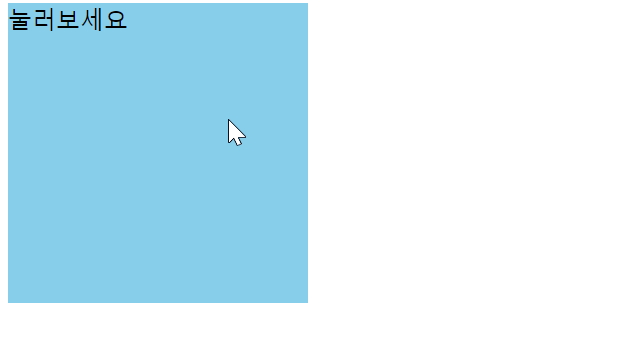
또한 Animate는 속성값의 크기를 증감하여 다양한 형태로 표현 가능하다.
주의 :: "+=150px" 안에 스페이스바(공백)가 들어가면 안됨!
$(document).ready(function(){
$("#box2").click(function(){
$("#box2").animate({
left: '250px',
height: '+=150px',
width: '+=150px'
});
});
});
<div id="box2" style="background-color:skyblue; width:200px; height:200px;position:absolute;">눌러보세요</div>
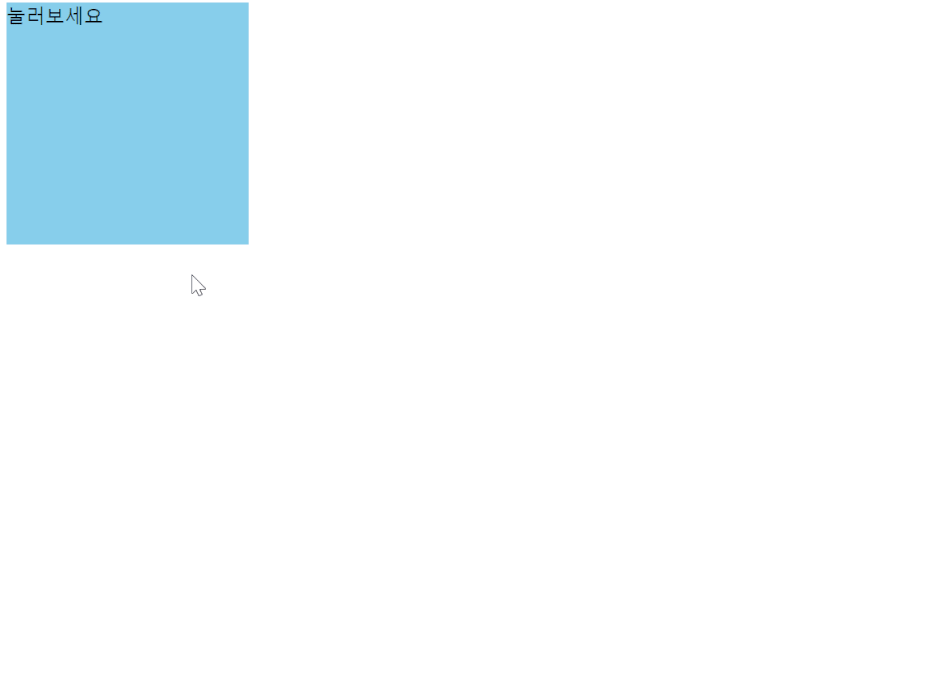
3. Animate toggle
속성을 toggle 로 하여 이벤트에 따라 상태를 계속 변경할 수 있다.
$(document).ready(function(){
$("#btn").click(function(){
$('#box').animate({
width: 'toggle'
});
});
});
<button id="btn">퐁</button>
<div id="box" style="background-color:pink; width:100px; height:100px; position:absolute;"></div>
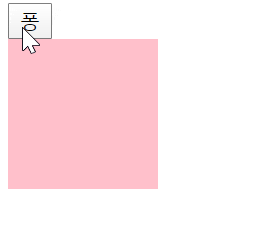
4. Animate 순차적으로 실행
선택자를 변수에 넣으면 여러 효과를 순차적으로 실행할 수 있다.
- 상하좌우 움직이기
$(document).ready(function(){
$("#btn").click(function(){
var div = $('#box');
div.animate({height: '300px', opacity: '0.4'}, "slow");
div.animate({width: '300px', opacity: '0.8'}, "slow");
div.animate({height: '100px', opacity: '0.4'}, "slow");
div.animate({width: '100px', opacity: '0.8'}, "slow");
});
});
<button id="btn">퐁</button>
<div id="box" style="background-color:pink; width:100px; height:100px; position:absolute;"></div>
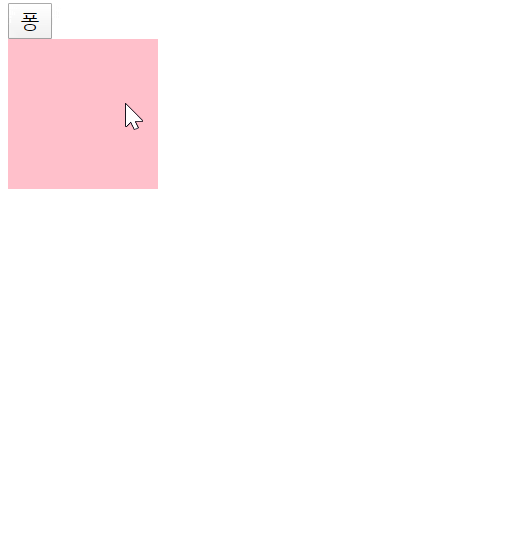
- div 이동 후 폰트 확대
$(document).ready(function(){
$("#btn").click(function(){
var div = $('#box');
div.animate({left: '100px'}, "slow");
div.animate({fontSize: '3em'}, "slow");
});
});
<button id="btn">퐁</button>
<div id="box" style="background-color:pink; width:100px; height:100px; position:absolute;">헤헿</div>
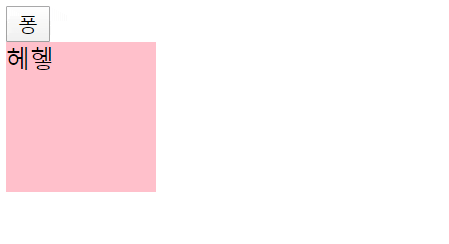
'JAVASCRIPT > JQUERY' 카테고리의 다른 글
slide (0) | 2022.08.28 |
---|---|
fadeIn - fadeOut (0) | 2022.08.28 |
hide - show (0) | 2022.08.28 |
Event (0) | 2022.08.28 |
Selector (0) | 2022.08.28 |